Opening a file in c
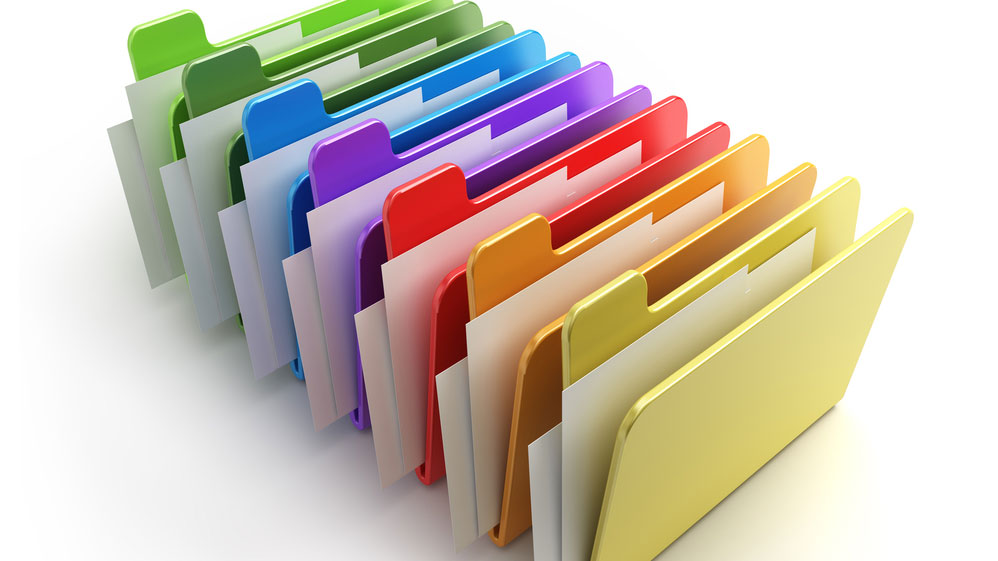
Opening a file in c
A file must be opened before any I/O operation can be performed on that file .
The process of establishing a connection between the program and the file is called opening the file
A structure named FILE Is defined in the file stdio.h that contains all information about the file like name , status , buffer size , current position , end of file status etc .
All these details are hidden from the programmer and the operating system takes care of all these things.
typedef struct { …………. …………… } FILE;
A file pointer is a pointer to a structure of type FILE . Whenever a file is opened , a structure of type FILE is associated with it , and a file pointer that points to this structure identifies this file .
The function fopen() is used to open a file
Declaration
FILE *fopen (const char *filename , const char *mode);
fopen() function takes two string as arguments , the first one is the name of the file to be opened and the second one is the mode that describes which operations ( read , write , append etc) are to be performed on the file .
On success , fopen() returns a pointer of type FILE and on error it returns NULL . The return value of fopen() is assigned to the pointer declared previously . For example
FILE *fp1 ,*fp2 ; Fp1 = fopen(“myfile.txt”,”w”); Fp2 = fopen(“myfile.dat”,”r”);
The name of the file is limited to FILENAME_MAX characters .
After opening the file with fopen() , the name of file is not used in the program for any operation on it .
whenever we have to performed any operation on the file we will use pointer returned by fopen() function .
so the original name is called external name but the file pointer associated with it is called internal name.
The second argument represent the mode in which file is opened . The possible modes are :
“w”(write)
– If the file does not exit then this mode create a new file and if the file is already exist then previous data is erased and new data entered is written to the file“a”(append)
- - If the file does not exit then this mode create a new file and if the file is already exist then new data is appended at the end of file . In this mode , the data existing in the file is not erased as in previous mode.“r”(read)
-- This mode is used to read existing data in the file . File must exist for this operation and data is not erased“w+”(write+read)
—This mode is similar to the write mode but here we can also read and modify the data . If the file does not exit then this mode create a new file and if the file is already exist then previous data is erased and new data entered is written to the file“r+”(read+write)
—This mode is similar to the read mode but here we can also write and modify the data .File must exist for this operation and data is not erased . Since we can add new data and modify existing data so this mode is also called update mode.“a+”(append+read)
—This mode is similar to the append mode but here we can also read the data stored in the file . If the file does not exit then this mode create a new file and if the file is already exist then new data is appended at the end of file . In this mode , the data existing in the file is not erased as in previous mode.To open a file in binary mode we can append ‘b’ to the mode , and to open the file in text mode ‘t’ can be appended to the mode .
But since text mode is the default mode , ‘t’ is generally omitted while opening files in text mode . For example-
“wb” - Binary file opened in write mode
“ab+” or “a+b” - Binary file opened in append mode
Errors in Opening Files
If an error occurs in opening the file , than fopen() return null . So we can check for any errors in opening by checking the return value of fopen() .FILE *fp ; fp = fopen(“myfile.dat”,”w”); if (fp == NULL) { printf(“error in opening file”); exit(1); }
Errors in opening a file may occur due to various reasons , for example-
1.If we try to open a file in a read or update mode , and the file does not exist or we do not have read permission on that file
2.If we try to create a file and there is no space on the disk , then we do not have write permission
3.Operating system limit the number of file that can be opened at a time and we are trying to open more file than that limit
Alert
We can give full pathname to open a file . Suppose we want to open a file DOS whose path is ”f:\file\myfile.dat” , then we have to write as –
fp = fopen(”f:\\file\\myfile.dat”, “r”);
Here we have used double backslash inside string is considered as an escape character , ‘\f’ and ‘\m’ are regarded as escape sequence if we use single backslash . In Unix , a single forward slash can be used
Never give the mode single quotes , since it is string not a character constant
fopen(”f:\\file\\myfile.dat”, ‘r’); //ERROR
You can Browse related article below for more information and program code related to file handling Does above is helpful , Post you views in comment
Comments
Post a Comment